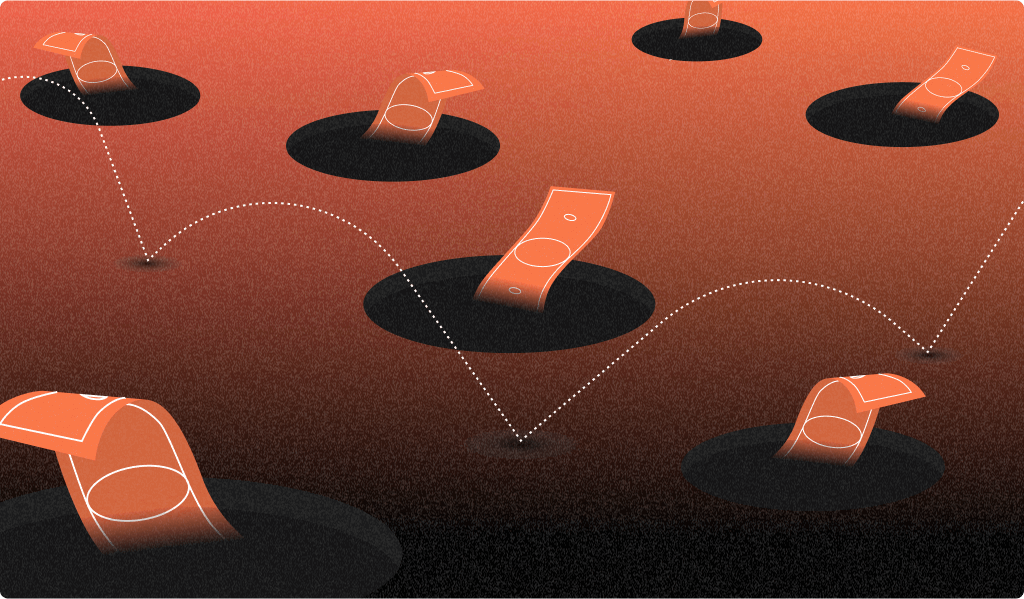
What is Loan Fraud?
Loan fraud is the intentional act of providing false or misleading information during the loan application process to obtain funds for which the applicant is not rightfully eligible. Misformation can range from exaggerating income and asset details to committing identity theft. Some applicants may even misrepresent the loan’s intended use, claiming it's for a seemingly less risky purpose, like home renovation, when it's actually for a more risky activity, such as gambling.
Another common method of loan fraud is applying for loans multiple times on the same lending platform under different names, aliases, or even fabricated identities. This technique is especially problematic because it manipulates the lender's system into treating each application as if it's from a unique, unrelated individual. This manipulation makes it significantly challenging for automated fraud detection mechanisms to flag the activity as suspicious, as each fraudulent application appears as an isolated case rather than part of a larger, coordinated fraud scheme
Why is it Important to Detect Repeat Loan Applications?
Identifying repeat loan applications is crucial for maintaining the integrity of the lending system and mitigating financial risk. There are several reasons to detect this type of fraud:
Increase in Loan Defaults
Undetected fraudulent repeat applications often result in the approval of high-risk loans that should otherwise be declined. These loans, secured through deceptive practices that manipulate the lender's risk assessment, are more likely to default, leading to financial loss for the lending institution and compromising its financial health.
Decreased Lender Effectiveness
Repeated loan applications with varying inputs distort the data lenders use for risk assessment. This data corruption undermines the reliability of the lender's risk assessment models as the data is skewed from the outcome of what is very likely a high-risk loan. As these models become less effective, the lender's ability to make sound financial decisions is compromised.
Regulatory and Legal Consequences
Failing to detect multiple fraudulent applications can also expose lenders to regulatory scrutiny and legal consequences. Regulatory bodies may impose fines or penalties for inadequate fraud detection measures. In extreme cases, poor oversight may even threaten the lender's operating license. This adds another reason for lenders to vigilantly monitor for signs of loan fraud, including multiple applications from the same individual.
Configuring Fingerprint Pro for Loan Risk Detection
Fingerprint Pro's Visitor Identification allows you to add extra signals and inputs to your loan risk assessment. This allows you to identify whether a returning visitor, even if anonymous, has previously applied for a loan. To accomplish this, you’ll need to create logic within your site that uses the visitor ID obtained from the Fingerprint Identification API. This should be combined with timestamped data and the loan inputs provided by the user. It’s important to thoughtfully construct the logic for detecting a repeat loan application and decide on the appropriate actions when a visitor is flagged for suspicious activity.
Suspicious Application Activity Logic
We suggest that when a visitor submits a loan application, the visitorId
and loan inputs are transmitted to your application server, where they are stored. With this data, you can determine if the visitor has recently submitted another loan application and compare the provided information. Below are some suggested logic rules for identifying repeat or fraudulent applications.
To begin, incorporate the Fingerprint Pro JavaScript agent into your webpage. Alternatively, consider using one of our libraries if your front end utilizes contemporary frameworks such as React.js or Angular.
// Initialize the agent.
const fpPromise = import("https://fpjscdn.net/v3/<your-public-api-key>").then(
(FingerprintJS) =>
FingerprintJS.load({
endpoint: "https://metrics.yourdomain.com",
})
);
For production deployments, we recommend routing requests to Fingerprint's APIs through your domain using the endpoint
parameter. This prevents ad blockers from disrupting identification requests and improves accuracy. We offer a variety of ways to do this, which you can learn more about in our guide on how to protect your JavaScript agent from ad blockers.
Next, make the fingerprint request and send the requestId
with the loan application details to your application server when the user submits their application.
async function onApplicationSubmit() {
// Collect browser signals and request visitor identification
// from the Fingerprint API. The response contains a `requestId`.
const { requestId } = await (await fpPromise).get();
// Send the loan details together with the `requestId`.
const loanData = {
firstName,
lastName,
monthlyIncome,
loanValue,
loanDuration,
requestId,
};
const response = await fetch("/api/assess", {
method: "POST",
body: JSON.stringify(loanData),
headers: {
"Content-Type": "application/json",
Accept: "application/json",
},
});
}
You should perform the following steps in your backend using data from the Fingerprint Pro Server API. If your backend logic is built on top of Node.js or any other popular server-side framework or language, you can use one of our Fingerprint Server API SDKs. Alternatively, one can use the Webhooks functionality.
Check that the requestId
you received is legitimate by hitting the Server API /events
endpoint.
const requestId = req.body.requestId;
const fingerprintServerApiUrl = new URL(
`https://api.fpjs.io/events/${requestId}`
);
const requestOptions = {
method: "GET",
headers: {
"Auth-API-Key": "<secret-api-key>",
},
};
const fingerprintServerApiResponse = await fetch(
fingerprintServerApiUrl.href,
requestOptions
);
// If there's something wrong with the provided data,
// the Server API will return a non-2xx response.
// We consider these data unreliable.
if (
fingerprintServerApiResponse.status < 200 ||
fingerprintServerApiResponse.status > 299
) {
// Handle the error internally, deny the application
// and/or flag the user for suspicious activity.
reportSuspiciousActivity(req);
return getDenialResponse(
res,
"Your loan application has been denied due to suspicious activity.",
"error"
);
}
const requestData = await fingerprintServerApiResponse.json();
const visitorData = requestData?.products?.identification?.data;
The Server API response must contain information about this specific identification request. If not, the request might have been tampered with and we don't trust this identification attempt.
// The returned data must have the expected properties.
if (requestData.error || visitorData?.visitorId == undefined) {
reportSuspiciousActivity(req);
return getDenialResponse(
res,
"Your loan application has been denied due to suspicious activity.",
"error"
);
}
Next, we can check for previous loan applications in a set amount of time. Your particular business rules will dictate an appropriate window for your use case, in our example, we are checking for repeat application submissions within the last 24 hours.
const timestampEnd = new Date();
const timestampStart = new Date(timestampEnd.getTime() - 24 * 60 * 60 * 1000);
// Find previous loan requests that were sent
// by this `visitorId` in the last 24 hours.
const previousLoanRequests = await LoanRequest.findAll({
where: {
visitorId: {
[Op.eq]: visitorData.visitorId,
},
timestamp: {
[Op.between]: [timestampStart, timestampEnd],
},
},
});
If you find previous loan applications within your selected period, you can then do some additional validation on the data that was submitted now and previously. For example, if the information about the person seeking the loan has changed, this could be a reg flag.
const { monthlyIncome, firstName, lastName } = JSON.parse(req.body);
if (previousLoanRequests.length) {
// Check if monthly income, first name, and last name
// are the same as in previous loan requests.
const hasValidFields = previousLoanRequests.every(
(loanRequest) =>
loanRequest.monthlyIncome === monthlyIncome &&
loanRequest.firstName === firstName &&
loanRequest.lastName === lastName
);
// Whoops, it looks like the data is not the same!
// You could potentially mark this user in your database
// as fraudulent, or perform some other actions.
// In our case, we return a warning.
if (!hasValidFields) {
return getWarningResponse(
res,
"We cannot approve your loan automatically since you have requested a loan with different personal details recently. We need to verify the provided information manually at this time. Please reach out to our agent with any questions.",
"warning"
);
}
}
At this point, you would want to do some additional checks, such as checking the timestamp of the visitor identifier request to make sure it is fresh and that the request came from an expected origin.
Finally, if all the checks are passed, continue with your loan assessment process, return the appropriate response, and store the visitorId
for future checks.
Explore Our Loan Fraud Demo
We built a fully open-source loan risk fraud demo to demonstrate the concepts above. Use this demo to see how to use Fingerprint Pro and simple logic rules to detect suspicious repeat loan applications. Feel free to view the live demo on CodeSandbox or jump into the GitHub repo for code. If you have any questions or want to learn more about how Fingerprint Pro can help you assess loan risk, please contact our support team.
FAQ
Risk assessment in lending evaluates the likelihood of a borrower defaulting, considering their financial history, credit score, and income. This determines loan approval and terms.
Use device fingerprinting to identify device-specific attributes such as IP address, browser type, and hardware configuration, revealing if the same device is used for multiple applications.
Device fingerprinting collects unique device data to identify and prevent fraudulent loan applications by detecting when the same device attempts to apply under different identities.