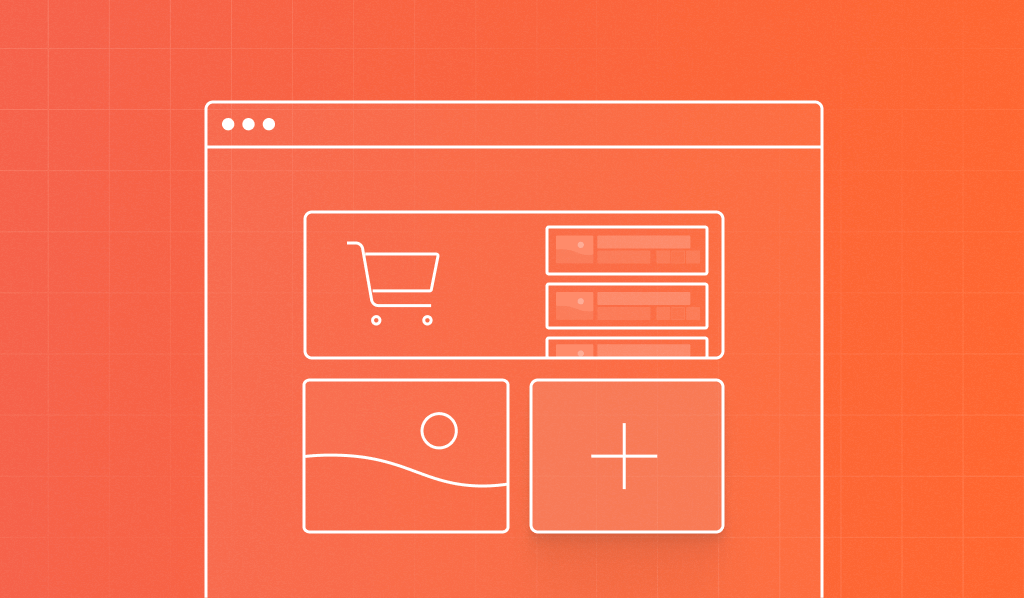
What Is User Personalization?
User personalization is the delivery of content and functionality that fits a user’s wants and needs without any effort required by the user.
The term "eCommerce personalization" relates to the set of practices in which an online store displays dynamic content based on customer data, such as demographics, intent, preferences, browsing history, previous purchases, and device usage—for instance, whether the customer is shopping on a mobile, tablet, or even a smartwatch. - Magnolia CMS
Why Is User Personalization Important?
Providing your users with a seamless, tailored experience helps with:
- Reducing bounce rates
- Improving conversion rates
- Increasing sales and revenue
How to Reduce Bounce Rate, Improve Conversions, and Increase Sales Through Personalization
Remember Light/Dark Mode Preferences
Offering a "dark mode" reduces bounce rates and retains visitors. Typically, a visitor’s site preferences are stored in session or local storage, which does not persist between browsing modes (standard and incognito).
Browser fingerprinting allows websites to remember anonymous visitor preferences regardless of a visitor’s browsing mode.
- When users toggle light/dark mode, save those preferences and a stable, unique identifier such as Fingerprint Pro's visitor ID.
- Look up the identifier on the app launch and set the light/dark mode preferences.
Demo: Storing Anonymous Browsing Preferences Using Browser Fingerprinting
Case Study: How Terra improved user engagement thanks to Dark Mode
Geolocation Suggestions
Travel applications can detect a user's location to provide a more tailored user experience. Recognizing where a user is accessing their application allows businesses to present them with timely, location-specific content or suggestions that can increase user engagement and satisfaction.
- Use the Geolocation API or Fingerprint Pro location identifiers to preset the closest airport as the default flight origin.
- Filter upcoming events based on proximity to the user’s location.
Stored Shopping Carts
Persistent items in a visitor’s shopping cart can reduce sales lost to shopping cart abandonment. Remembering shopping carts for days or weeks can also decrease checkout time for return visitors.
- Save a list of items associated with a visitor alongside Fingerprint Pro's visitor ID.
- When visiting the shopping cart, pull the list of items by the visitor ID.
Purchase and Browsing History
Saving product purchases and browsing history can help drive sales from return visitors. Typical cookie-based solutions do not work when visitors switch from regular to private browsing mode (or vice versa). We can solve this problem with browser fingerprinting.
- Use Fingerprint Pro to get a visitor ID and store it at checkout, return purchase history to the logged-out user when they return and their visitor ID is a match.
- Fingerprint each session, save browsed items with the visitor ID, and display the list when a visitor returns.
Article: 56% of shoppers are more likely to return a site that recommends products
E-Commerce Promotions
Running e-commerce promotions without user verification is risky and prone to abuse. Traditional verification methods require signing up for email or phone number marketing lists. Fortunately, we can streamline the verification process without user input. A stable and unique identifier is great for assigning promotions to anonymous visitors and preventing duplicate promotion abuse. Furthermore, promotions can be uniquely applied based on visitor behavior, i.e., return visits.
- Log visits with Fingerprint Pro's visitor ID and reward visitors with free shipping after a certain number of visits to a landing page.
- Offer limited-time promo codes for return visitors based on a matching visitor ID after numerous cart abandonments.
How to Increase Sales Through Personalization with Fingerprint Pro
Fingerprint Pro provides a unique identifier for every visitor to your website collected behind the scenes anytime someone performs a specific action with our JavaScript fingerprinting agent installed. Since the client-side data might be forged, Fingerprint Pro also provides tools for validating these identifiers sent by your front end. As a result, you can provide your users with a tailored experience between incognito and normal mode, even without cookies.
Since you know your product and business landscape best, it’s up to you to decide how to configure personalization workflows to utilize the visitor ID to improve user experience. When determining how and when to use personalization, it is helpful to consider user expectations, particularly when comparing normal and incognito browsing modes. Below, we have described some steps and best practices to use as a starting point for your custom solution.
Configuring Fingerprint Pro for Personalization Use Cases
We recommend that when a new user requests your site content, the visitorId
is sent to your application server. You can use this data to return personalized content such as search history, customized user interfaces, or even the user’s shopping cart.
First, you obtain a visitorId
from Fingerprint Pro's Identification API. Then, for the specific content that should be personalized, such as shopping cart, search queries, or dark mode, send these pieces of information within your request, persist necessary data if needed, and provide personalized content. Here are a few steps to provide these features to your users.
You need to add the Fingerprint Pro JavaScript agent to your webpage. Alternatively, if your front end uses modern frameworks such as React.js or Angular, you can use one of our libraries instead.
// Initialize the agent.
const fpPromise = import("https://fpjscdn.net/v3/<your-public-api-key>").then(
(FingerprintJS) =>
FingerprintJS.load({
endpoint: "https://metrics.yourdomain.com",
})
);
// Once you need a fingerprinting result, get and store it.
// Typically, on page load or button click.
const fp = await fpPromise;
const result = await fp.get();
For production deployments, we recommend routing requests to Fingerprint's APIs through your domain using the endpoint
parameter. This prevents ad blockers from disrupting identification requests and improves accuracy. We offer a variety of ways to do this, which you can learn more about in our guide on how to protect your JavaScript agent from ad blockers.
Personalized Shopping Cart Content
Send the user's visitorId
to your servers when requesting shopping cart content.
const response = await fetch(`/api/preferences`, {
method: "POST",
body: JSON.stringify({ visitorId: result.visitorId }),
headers: {
"Content-Type": "application/json",
Accept: "application/json",
},
});
To get the user’s cart content on the server side, use code similar to the following.
const visitorId = req.body.visitorId;
// Returns cart items for the given visitorId.
const cartItems = await UserCartItem.findAll({
where: {
visitorId: {
[Op.eq]: visitorId,
},
},
order: [["timestamp", "DESC"]],
include: Product,
});
return res.status(200).json({
data: cartItems,
size: cartItems.length,
});
To add an item to the user's shopping cart, update cart items for the given visitorId
.
// Adds an item to the cart for the given `visitorId`.
const [cartItem, created] = await UserCartItem.findOrCreate({
where: {
visitorId: {
[Op.eq]: visitorId,
},
productId: {
[Op.eq]: productId,
},
},
defaults: {
visitorId,
count: 1,
timestamp: new Date(),
productId,
},
});
if (!created) {
cartItem.count++;
await cartItem.save();
}
return res.status(200).json({
data: cartItem,
});
Personalized Search History
Send the user's visitorId
when getting search history to pull up their recent searches.
// Get user search history for a given `visitorId`.
const history = await UserSearchHistory.findAll({
order: [["timestamp", "DESC"]],
where: {
visitorId: {
[Op.eq]: visitorId,
},
},
});
return res.status(200).json({
data: history,
size: history.length,
});
Similarly, to update the user's search history, use the following code to persist or retrieve the search history for the specific visitorId
.
// Persist search query for a given `visitorId`.
const existingHistory = await UserSearchHistory.findOne({
where: {
query: {
[Op.eq]: query,
},
visitorId: {
[Op.eq]: visitorId,
},
},
});
if (existingHistory) {
existingHistory.timestamp = new Date().getTime();
await existingHistory.save();
return;
}
await UserSearchHistory.create({
visitorId,
query,
timestamp: new Date().getTime(),
});
User's Preferences and Dark Mode
Additionally, you can get or set other user preferences, such as user interface dark mode.
To get or set user preferences according to their visitorId
:
// Update user preferences for given `visitorId`.
const { hasDarkMode } = JSON.parse(req.body);
const hasDarkModeBool = Boolean(hasDarkMode);
const [userPreferences, created] = await UserPreferences.findOrCreate({
where: {
visitorId: {
[Op.eq]: visitorId,
},
},
defaults: {
visitorId,
hasDarkMode: hasDarkModeBool,
},
});
if (!created) {
userPreferences.hasDarkMode = hasDarkModeBool;
await userPreferences.save();
}
return res.status(200).json({
data: userPreferences,
});
Trusting the Information Provided by the Client Side
Since data such as visitorId
and requestId
provided by the client side might be spoofed, it's good practice to check and validate provided data with our Server API or Webhooks. This is not critical for remembering dark mode or shopping cart preferences but is highly recommended for more sensitive use cases. Take a look at Protecting from client side tampering in our documentation. For most personalization use cases, it's more important for your website to gracefully fall back to the default content if the provided visitor ID is invalid.
Explore Our Personalization Demo
We have a Personalization demo to demonstrate the above concepts. Use the demo to visualize using Fingerprint Pro and simple logic rules to provide a tailored experience to your users. If you want to explore code, check our interactive CodeSandbox demo or open-source GitHub repository. If you have any questions, please feel free to reach out to our support team.
FAQ
When a past visitor returns a website can tailor its content to match their preferences and past activity, such as using preferred theme settings or showing previously viewed products.
Personalization enhances user experience, encouraging longer visits and repeat traffic, and can increase conversion rates by displaying relevant content or products.
Even though users have not logged in, device fingerprinting can help identify repeat visitors. Store this identifier along with their preferences so you can personalize their experience, even for anonymous users.