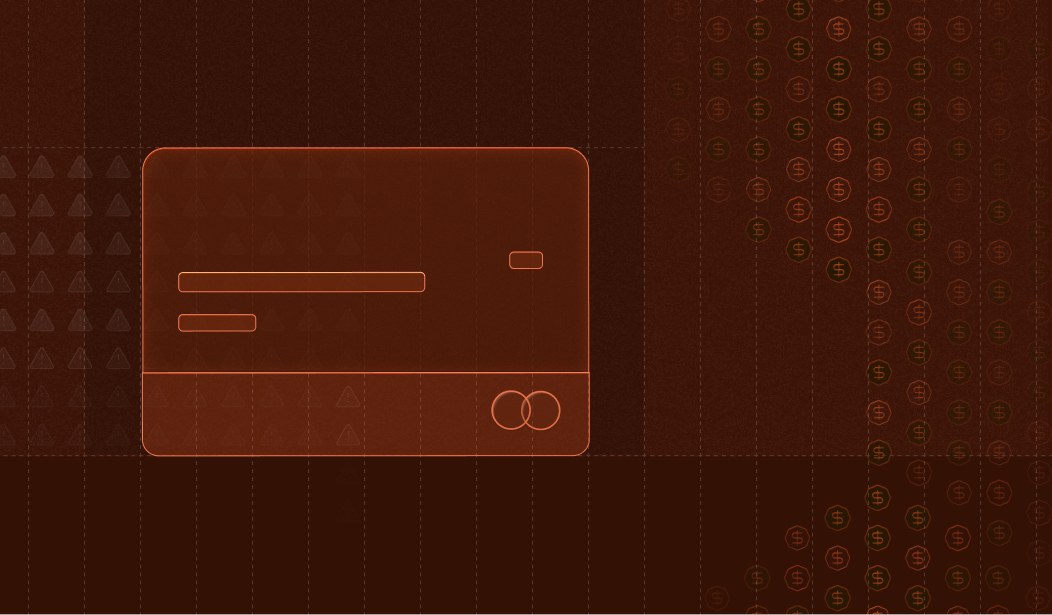
Payment fraud, AKA the unauthorized use of payment methods, lurks in all kinds of digital transactions, from e-commerce platforms to subscription services, chipping away at bottom lines and eroding customer trust. With online payment fraud losses expected to climb from $41 billion in 2022 to $91 billion in 2028, businesses are up against an increasingly sophisticated and costly battle.
The damage isn’t just financial. Fraud can trash reputations, frustrate customers, and drain resources as businesses scramble to chase down and investigate fraudulent activity. And fraudsters have no shortage of methods, from using stolen payment details to account takeovers and system exploits.
In this article, I’ll break down common types of payment fraud, explore its impact on businesses and consumers, and share steps to keep your business and customers protected.
Common payment fraud varieties
Payment fraud comes in many forms, each cleverly designed to exploit vulnerabilities in digital platforms and human behavior. Let’s dive into some of the most common offenders that force businesses to strike a balance between keeping fraudsters at bay and ensuring a seamless experience for their customers.
- Card-not-present (CNP) fraud: The reigning champion of payment scams. This is when a fraudster uses stolen credit or debit card details to shop online where no physical card is required. Fraudsters may get credit card info from a data breach, physical card skimming, or brute-force tactics like card cracking and testing.
- Account takeover (ATO): When fraudsters hijack a user’s account, usually by phishing or using leaked credentials, to make purchases or transfer funds. Many e-commerce sites allow customers to store their payment information for fast checkouts. Unfortunately, what’s convenient for the customer is equally convenient for the fraudster.
- Friendly or chargeback fraud: Chargeback fraud is when customers dispute legitimate transactions with their bank, insisting they never received the goods or claiming their card was used without their consent. The result? They walk away with both the goods and the refund, pulling off a win-win for themselves and a lose-lose for the merchant.
- Identity theft: In the context of payment fraud, this is when fraudsters “play pretend” with someone else’s personal information to open accounts, apply for credit, or make unauthorized purchases. For example, a thief gets hold of a victim’s Social Security number and address, opens a shiny new credit card, and maxes it out on a shopping spree.
- Coupon and signup abuse: When fraudsters exploit discounts, promo codes, or rewards to game the system for freebies. Whether it’s creating fake accounts to snag endless free trials and referral bonuses or spinning up duplicate emails to milk that 25%-off new customer discount, these schemes take advantage of promotions intended for legitimate customers.
The hidden cost of payment fraud
Payment fraud doesn’t just cost billions in chargebacks, refunds, and stolen goods — it can also drag businesses down with legal fees, investigation costs, and wasted time. The damage to your reputation is just as painful. Customers who see your site as unsafe may abandon your business permanently and warn others to stay away. Your payment partners won’t be too thrilled either, and too much fraud could lead to higher fees or even the loss of the partnership entirely.
To make matters worse, your team is forced to spend countless hours fighting fraud instead of focusing on growth. And if you tighten security measures too much, you risk slowing legitimate sales and frustrating loyal customers. It’s a domino effect of financial losses, operational headaches, and eroded trust that can leave your business struggling to keep up.
Tackling payment fraud with Fingerprint
Being able to recognize return users and understand their device characteristics is a game-changer for businesses fighting payment fraud. By analyzing things like browser settings, hardware details, and network configurations, you can spot red flags and prevent fraud before it even happens.
Fingerprint is an industry leader in device intelligence, offering the highest accuracy in recognizing returning visitors. Each device or browser that visits your site or mobile app gets a unique visitor ID that stays the same, even if the user clears cookies, uses a VPN, or switches to incognito mode.
Plus, Fingerprint’s Smart Signals dive deeper, giving you insights into suspicious behavior and traits that can give you a clue about a visitor’s intent, even if it’s their first time on your site. We can tell you if bot activity is detected, if the visitor has tampered with their browser, or if their device attributes look suspicious compared to your average user. Fingerprint gives you the data you need to block the fraudsters hiding in your traffic while keeping things smooth for your legitimate customers.
Fingerprint implementation examples
To start preventing payment fraud with Fingerprint data, you first need to identify a user visiting your site or app. Once you have the Fingerprint visitor ID, you can weave it into any part of your fraud detection and prevention workflows. Below, we provide some steps and best practices that can serve as a starting point for your custom solution.
Getting a visitor ID
The first step is always identifying the visitor and retrieving their visitor ID. To do this, you’ll need to request identification from Fingerprint on the front end. You can easily implement this using one of our SDKs and libraries for your preferred framework or language. Don’t forget to grab your public and secret API keys from the Fingerprint dashboard.
Make sure to load Fingerprint as soon as possible and then request identification when you need it. In this example, the identification request happens when an order is placed.
// Initialize the agent as soon as possible
const fpPromise = import('https://fpjscdn.net/v3/PUBLIC_API_KEY')
.then(FingerprintJS => FingerprintJS.load())
async function placeOrder(orderDetails) {
// Make an identification request when you need it.
const fp = await fpPromise;
const result = await fp.get();
const { requestId } = result;
// Include the requestId with the order details
const orderPayload = {
...orderDetails,
requestId,
};
const requestOptions = {
method: "POST",
body: JSON.stringify(orderPayload),
headers: {
"Content-Type": "application/json",
Accept: "application/json",
},
};
// Send the order payload to your server
const response = await fetch("/api/place-order", requestOptions);
// ... additional order logic
}
You might have noticed we haven’t used the visitor ID just yet. When you make an identification request, you’ll get both a visitor ID and a request ID. It’s a best practice to use the request ID on your server to pull the full details of the event — like bot activity, VPN usage, the real visitor ID, and more. This helps prevent tampering since client-side data can’t always be trusted.
In this example, we’re using the Node SDK to retrieve data on the server. From here on, all steps should be handled server-side, where you can perform logic checks and process payments securely.
import {
FingerprintJsServerApiClient,
Region,
} from "@fingerprintjs/fingerprintjs-pro-server-api";
const client = new FingerprintJsServerApiClient({
apiKey: "SECRET_API_KEY",
region: Region.Global,
});
// Get the full details of the visitor identification event
const identificationEvent = await client.getEvent(requestId);
To ensure the most accurate identification, make sure to prevent ad blockers from blocking your requests. For more detailed instructions on setting up Fingerprint to identify visitors on your site or app, check out our documentation.
Preventing card cracking and testing
Once you have the visitor ID and the identification details, there are several ways to use them to prevent payment fraud. One way is to prevent bots from making payments.
Card cracking and testing involve fraudsters using automated tools to try thousands of stolen or generated payment card combinations. They may have real partial card information or be starting from scratch. The first line of defense is to use Fingerprint’s bot detection to block these automated tools from even attempting payments.
The bot detection signal returns good
for known bots like search engines, bad
for malicious bots, and notDetected
if no bots were detected.
// Prevent bots from making purchases
if (identificationEvent.products?.botd?.data?.bot?.result !== "notDetected") {
return {
success: false,
error: "Purchase is blocked.",
};
}
Stop past offenders
When fraudsters manage to pull off a successful fraudulent payment, it’s important to ensure they don’t get a chance to try again. By storing the visitor ID of a fraudster along with payment or order details, you can build a blocklist to stop them from coming back for more. You can use this for all types of fraud. For example, a single chargeback might not be much, but a bunch of them in a short time is very likely fraud.
const [rows] = await db.execute(
`SELECT COUNT(*) AS chargebackCount
FROM payment_attempts
WHERE visitor_id = ?
AND is_chargeback = 1
AND timestamp > NOW() - INTERVAL 30 DAY`,
[visitorId]
);
const chargebackCount = rows[0]?.chargebackCount;
if (chargebackCount > 3) {
return {
success: false,
error: "Purchase blocked.",
};
}
Similar logic can be used for failed payments or visitors who were later found to be using stolen payment methods. If the above checks have passed, continue with the purchase and store the visitorId
for future payment verifications.
Challenge suspicious activity
Sometimes, fraud isn’t as clear-cut as the examples above, and instead of blocking a payment outright, you can choose to take different precautionary actions. For example, you can use Fingerprint’s Suspect Score to quickly assess a user’s risk level. This handy weighted number gives you a quick look at the user’s risk level, based on the global probability of their suspicious attributes. The higher the score, the more suspicious the visitor.
When you identify a potentially risky user trying to make a payment or purchase, you can ask for a little more proof they’re not a fraudster, such as second form of authentication, email verification, or an one-time passcode sent via SMS. These extra measures help ensure that the transaction is legitimate without causing unnecessary friction for legitimate users.
// Detect the risk level of the visitor
if (identificationEvent.products?.suspectScore?.data?.result > 15) {
return {
success: false,
error: "Suspicious activity detected. Verification is required.",
action: "sendOTP", // Trigger your specified action for verification.
};
}
Explore our payment fraud prevention demo
Payment fraud is a relentless and ever-evolving headache that costs businesses more than just money, it can wreck reputations, annoy customers, and drain your time and resources. But with the right tools, like Fingerprint’s highly accurate visitor identification and real-time device intelligence, you can outsmart fraudsters without making life harder for your real customers.
We’ve built a payment fraud prevention demo to show you exactly how this works in action. Use it to see how Fingerprint’s data, combined with your own logic rules, can protect your payment forms. Want to dig into the code? Check out our interactive CodeSandbox demo or browse the open-source GitHub repository. And if you have questions, don’t hesitate to contact our support team.
FAQ
Payment fraud involves unauthorized transactions and financial theft, often through stolen credit card details or identity theft, leading to chargebacks and revenue loss for businesses.
Implementing multi-factor authentication, monitoring for unusual transaction patterns, and maintaining a blocklist of known fraudulent devices can further reduce payment fraud risks.