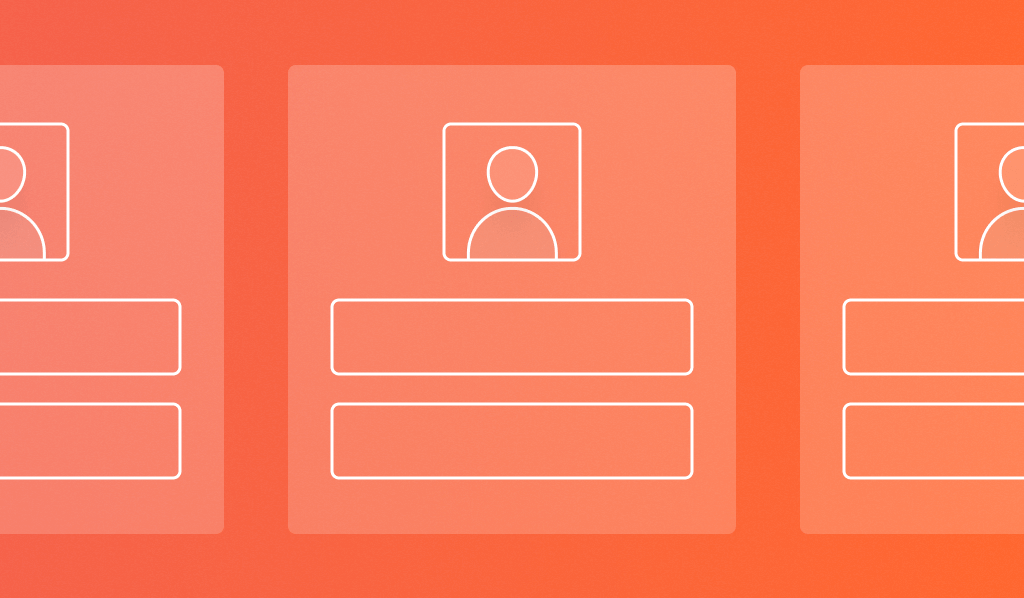
Dealing with fraudsters creating multiple fake accounts can be a headache for online businesses. These fraudsters can exploit services, drain resources, and compromise user security. Imagine an online marketplace where fraudsters create numerous fake accounts to post bogus listings or manipulate product reviews.
Or picture a promotional campaign offering a free trial or discount for new accounts, where these fraudsters repeatedly sign up to exploit the offer, depriving genuine customers of the opportunity. A social platform scenario is also concerning, where fraudsters could use multiple fake accounts to spread misinformation or harass users.
In this article, you'll learn what account creation fraud is, its impact on businesses, and prevention methods.
What is account creation fraud?
Account creation fraud occurs when fraudsters repeatedly create fake accounts on a digital platform. Fraudsters employ this tactic for various nefarious purposes, such as unfairly claiming new user benefits like discounts and trial subscriptions, posting spam or malicious content to affect public opinion, or coordinating harassment campaigns.
It's also commonly used to skew polls, contests, or rating systems through artificially inflated voting or to bypass purchasing restrictions on products to buy out stock and resell at higher prices.
How does account creation fraud impact businesses?
Beyond the operational challenges, this new account fraud can result in significant financial losses for businesses, degraded user experiences due to spam and low-quality content, coordinated harassment campaigns against legitimate users, and potential security vulnerabilities.
Some examples of its adverse effects on businesses include:
- Resource Drain: Fraudsters often create multiple accounts to take advantage of free trials or new user discounts. For example, a streaming service offering a month-long free trial might find fraudsters repeatedly signing up to avoid paying for the service, leading to significant revenue loss and increased operational costs in managing these fraudulent accounts.
- Inaccurate Quality Data: Fake accounts can manipulate ratings and reviews, harming the reputation of products or services. For instance, a fraudster might use multiple accounts to post negative reviews on a competitor's product page, driving away potential customers and tarnishing the brand's image.
- Poll Manipulation: Fraudsters use numerous accounts to influence the outcome of contests or polls. For instance, in a user-voted product design contest, a fraudster could create numerous accounts to vote for a particular entry, skewing the results in favor of an undeserving winner. Undermining the fairness of the competition, damaging the company's credibility, and discouraging engagement in future events.
- Inventory Exploitation: In e-commerce, fraudsters might bypass purchase limits on popular or limited-edition items to hoard products and resell them at a higher price. Over-purchasing deprives genuine customers of the chance to purchase these products and can disrupt inventory management and pricing strategies.
- Degraded User Experience: Multiple accounts can spread spam or malicious content or engage in coordinated harassment campaigns against specific users or groups. This content harms individuals and can create a toxic environment. Businesses must find ways to permanently ban these spam accounts or risk malicious content driving users away from the platform.
How do you detect and prevent fraudulent signups?
Preventing account creation fraud is essential for preserving the integrity and trustworthiness of online platforms. As fraudsters use more sophisticated and automated methods, businesses must adopt robust, multi-layered defenses to secure their websites.
Counter-measures like CAPTCHA challenges and two-factor authentication can fortify defenses against fake account creation methods. Using a variety of strategies can create multiple reinforcing layers of security. A few examples of these strategies include:
- CAPTCHA Challenges: Implement CAPTCHA challenges during signup to block automated bots from creating accounts en masse. Distinguishing between human users and bots can drastically slow fake account creation; however, CAPTCHAs degrade the user experience.
- Multi-Factor Authentication (MFA): Incorporating MFA at signup adds a layer of verification, making it more cumbersome for fraudsters to create multiple accounts, especially on a large scale. Examples include emailing magic links, sending OTPs (one-time passwords), or using authenticator applications.
- Using Cookies: Cookies can help identify returning browsers, distinguishing between legitimate users and potential fraudsters. Unfortunately, users can easily manipulate or clear them, reducing their reliability, and they require careful consideration of privacy laws and user consent.
- Device and Browser Fingerprinting: Leveraging device and browser fingerprinting can identify devices, allowing websites to block multiple account creation attempts from the same device. Fingerprinting can repeatedly identify the same device or browser without relying on cookies, making it much harder for fraudsters to abuse signup incentives.
- Analyze User Behavior: Employing machine learning algorithms to analyze user behavior can help identify patterns indicative of fraudulent activity, such as rapid completion of signup forms, sequential username creation, and privacy tools like VPNs. Additionally, unusual navigation patterns, such as abnormal browsing speed, unusual or exact click patterns, or failure to engage with site content, can signal potential fraud attempts.
- Limit IP Address Signups: Monitor and restrict the number of accounts created from a single IP address within a specific timeframe. These restrictions can prevent mass account creation from the same source, though they may not catch fraudsters using VPNs or proxy servers. Additionally, it can inadvertently block legitimate users sharing an IP address, such as those at universities or workplaces.
Preventing fraudulent signups with Fingerprint
Using browser and device fingerprinting stands out as a highly effective method in the fight against account creation fraud. This technique involves collecting and analyzing characteristics of a user's device and browser, such as the operating system, screen resolution, installed fonts, and more, to create a distinctive fingerprint or identifier. Unlike IP addresses or cookies, which fraudsters can easily change or mask, a stable browser or device fingerprint offers a more persistent means of identifying devices over time.
Fingerprint is a device intelligence platform that provides 99.5% accurate identifiers for browsers and mobile devices. Our identifiers are stable for months or years. They can accurately recognize a returning browser or device 99.5% of the time, even when the user uses a VPN or is in incognito mode.
We also provide Smart Signals for detecting potentially suspicious behaviors like browser tampering, VPN use, or whether the user is a bot to help companies develop strategies to protect their websites from fraudsters.
Our Identification API allows you to recognize a returning visitor, even if anonymous, to determine if they have previously signed up for an account. To do this, create logic within your site to obtain a visitor identifier from Fingerprint and store it with your account creation data. You can then access this data when a user attempts to create an account to see how many accounts they have already made. You then have the ability to decide how to proceed based on your own business rules.
The following tutorial will go through an example of a way to implement such logic to prevent account creation fraud using Fingerprint.
Obtaining a visitor identifier
The first step in preventing account creation fraud is to obtain the visitor identifier. We suggest you request the identifier when a visitor submits an account creation form.
To begin, incorporate the Fingerprint Pro JavaScript agent into your webpage. Alternatively, consider using one of our libraries if your front end utilizes popular frameworks like React.js or Svelte.
// Initialize the agent.
const fpPromise = import("https://fpjscdn.net/v3/PUBLIC_API_KEY").then(
(FingerprintJS) =>
FingerprintJS.load({
endpoint: "https://metrics.yourdomain.com", // Optional
})
);
We recommend routing requests to Fingerprint's APIs through your domain for production deployments using the optional endpoint parameter. This routing prevents ad blockers from disrupting identification requests and improves accuracy. We offer a variety of ways to do this, which you can learn more about in our guide on how to protect your JavaScript agent from ad blockers.
Next, request the visitor identifier when the visitor submits a new user form and send the requestId
with the potential new account details to your application server.
async function onNewUserSubmit() {
// Collect browser signals and request visitor identification
// from the Fingerprint API. The response contains a requestId.
const { requestId } = await (await fpPromise).get();
const username = document.getElementById("username").value;
const password = document.getElementById("password").value;
// Send the new account details together with the requestId.
const userData = {
username,
password,
requestId,
};
const response = await fetch("/api/user", {
method: "POST",
body: JSON.stringify(userData),
headers: {
"Content-Type": "application/json",
Accept: "application/json",
},
});
}
Validating the visitor identifier
You should perform the following steps in your backend using the Fingerprint Server API. You can use one of our SDKs if your backend logic uses Node.js or any other popular server-side framework or language.
To access the Server API, you must use your Secret API Key. You can obtain yours from the Fingerprint dashboard at Dashboard > App Settings > API Keys.
Before using the identification data from the front end, it's good to check that the requestId
you received is legitimate by hitting the Server API /events endpoint. This endpoint allows you to get additional data for an identification request. First, get the data from the server.
app.post('/api/user', async (req, res) => {
const { username, password, requestId } = req.body;
const fpServerEventUrl = `https://api.fpjs.io/events/${requestId}`;
const requestOptions = {
method: 'GET',
headers: {
'Auth-API-Key': 'SECRET_API_KEY',
},
};
// Get the detailed visitor data from the Server API.
const fpServerEventResponse = await fetch(fpServerEventUrl, requestOptions);
The Server API response must contain information about this specific identification request. If the request for this information fails or doesn't include the visitor information, the request might have been tampered with, and we don't trust this identification attempt.
// If there's something wrong with the provided data,
// the Server API will return a non-2xx response.
if (fpServerEventResponse.status < 200 || fpServerEventResponse.status > 299) {
// Handle the error internally, deny the new user creation,
// and/or flag the new user for suspicious activity.
reportSuspiciousActivity(req);
return getDenialReponse(
res,
"New user could not be created due to suspicious activity."
);
}
// Get the visitor data from the response.
const requestData = await fpServerEventResponse.json();
const visitorData = requestData?.products?.identification?.data;
// The returned data must have the expected properties.
if (requestData.error || visitorData?.visitorId == undefined) {
reportSuspiciousActivity(req);
return getDenialReponse(
res,
"New user could not be created due to suspicious activity."
);
}
A fraudster may be using an automated bot to try and programmatically create a large amount of new accounts. Check if the visitor is a human or a bot.
// Get the bot detection data from the response.
const botDetection = responseData?.products?.botd?.data;
// Determine if the user is a human or a bot.
if (botDetection?.bot?.result === "bad") {
reportSuspiciousActivity(req);
// Optionally: saveAndBlockBotIp(botDetection.ip);
return getDenialReponse(
res,
"New user could not be created due to automated bot detection."
);
}
A fraudster might have acquired a valid request ID via phishing. Check the freshness of the identification request to prevent replay attacks.
// The identification event must be a maximum of 3 seconds old.
if (new Date().getTime() - visitorData.created_at > 3000) {
reportSuspiciousActivity(req);
return getDenialReponse(
res,
"New user could not be created due to suspicious activity."
);
}
We also want to check if the new account request comes from the same IP address as the identification request.
// This is an example of obtaining the client's IP address.
// In most cases, it's a good idea to look for the right-most
// external IP address in the list to prevent spoofing.
if (req.headers["x-forwarded-for"].split(",")[0] !== visitorData.ip) {
reportSuspiciousActivity(req);
return getDenialReponse(
res,
"New user could not be created due to suspicious activity."
);
}
Next, check if the identification request comes from a known origin and if the request's origin corresponds to the origin provided by the Fingerprint Pro Server API. Additionally, one should also set the Request Filtering in the Fingerprint dashboard.
const ourOrigins = ["https://yourdomain.com"];
const visitorDataOrigin = new URL(visitorData.url).origin;
// Confirm that the request is from a known origin.
if (
visitorDataOrigin !== req.headers["origin"] ||
!ourOrigins.includes(visitorDataOrigin) ||
!ourOrigins.includes(req.headers["origin"])
) {
reportSuspiciousActivity(req);
return getDenialReponse(
res,
"New user could not be created due to suspicious activity."
);
}
Checking for multiple signups
Once the visitor identifier from the front end is validated, the next step is to check if this visitor has made multiple new accounts. We recommend using a specific timeframe and a threshold of new accounts that work with your business use case.
Query your data storage for any user accounts associated with the visitor identifier created in your specific time range. You can then decide how to handle the new account request, such as denying the creation or requesting additional verification.
// Gets all accounts created by the visitor in the last 30 days.
const countOfUsersForVisitorId = await db.query(
"SELECT COUNT(*) AS count FROM users WHERE visitor_id = ? AND created_at > ?",
[visitorData.visitorId, new Date().getTime() - 30 * 24 * 60 * 60 * 1000]
);
// Check if the visitor has created more than 5 accounts
// in the last 30 days. This threshold should be based
// on your business needs.
if (countOfUsersForVisitorId.count > 5) {
reportSuspiciousActivity(req);
return getDenialReponse(
res,
"New user not created, too many accounts created from this device."
);
}
If the visitor passes all the above validation and new user account checks, continue creating the new account and return a successful response.
// If all the above checks pass, create the new user
// and save the visitor identifier along with it.
// Note: this is a simple example and does not
// include proper password handling.
await db.query(
'INSERT INTO users (username, password, visitor_id, created_at) VALUES (?, ?, ?, ?)',
[username, password, visitorData.visitorId, new Date().getTime()]
);
return getSuccessReponse(res, 'New user created!');
});
Conclusion
Stopping multiple fake account signups is essential for keeping online platforms secure and trustworthy. With defenses against signup fraudsters, companies can retain resources and present a great user experience.
Determined fraudsters can bypass basic protections like IP blocking and email verification. However, tools like CAPTCHA can be annoying and add friction to legitimate users. Balancing strong protections with an excellent user experience requires using more sophisticated techniques like browser and device fingerprinting to recognize them accurately.
Contact our team if you'd like to learn more about Fingerprint's 99.5% accurate visitor identification, or start a free trial to see it in action for yourself.
FAQ
Account creation fraud involves fraudsters creating multiple accounts on an online platform to exploit benefits or disrupt services. It challenges businesses by draining resources, compromising user trust, and degrading user experiences.
To detect account creation fraud, monitor for rapid, sequential signups and repetitive use of the same IP address. Use cookies or fingerprinting to identify devices or implement CAPTCHA challenges to block automated bots.
Multi-accounting involves creating multiple legitimate accounts by a single user on a platform to separate interests, bypass restrictions or gain unfair advantages, often violating terms of service. Account creation fraud involves creating fake accounts using stolen or fake identities, typically for malicious purposes like financial fraud or spreading misinformation.