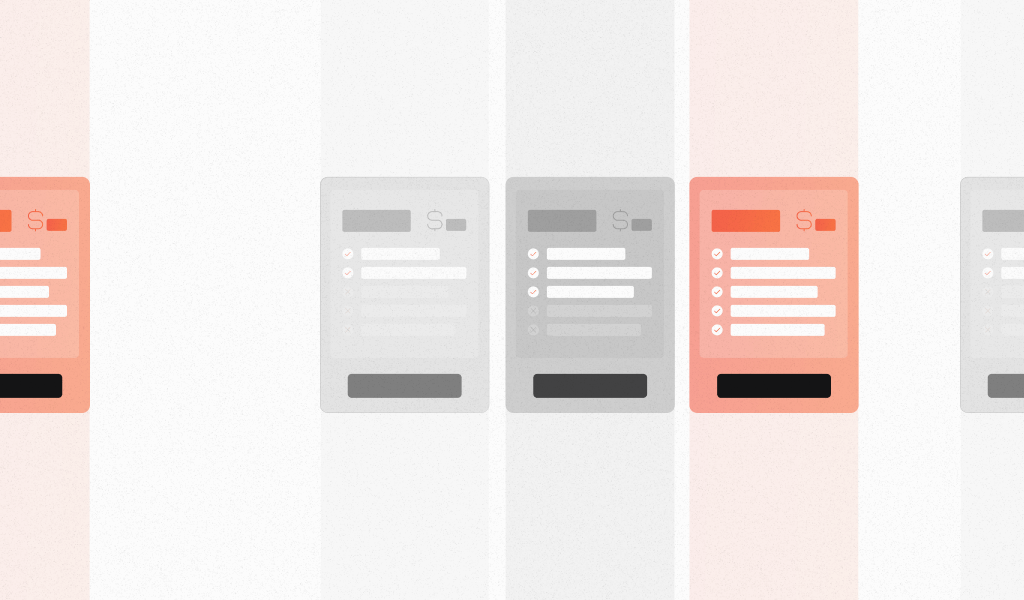
You've likely encountered paywalls while browsing the web for interesting content or the latest news. As annoying as they may seem from a reader's point of view, paywalls play an essential role in allowing publishers to monetize their content. Without a direct revenue stream from paying customers, publishers rely solely on advertising, which may not be sustainable and is annoying for readers.
However, some users find clever ways around these walls, skirting the rules to access restricted content without paying. This article will explore paywalls, why publishers implement them, what paywall evasion entails, and how publishers can protect their content and enforce access rules.
What is a paywall?
A paywall is a method to restrict users from accessing online content, requiring users to pay or subscribe to view it. Online newspapers, magazines, and content providers commonly employ this model to protect high-value content and establish a direct revenue stream from their readers. When visitors land on a page, the paywall checks if they have the necessary permissions, usually if they've paid or subscribed. If not, the content remains inaccessible, often accompanied by a prompt for payment or subscription.
For users willing to pay, paywalls enable unencumbered access while supporting the hard work that goes into quality journalism and content creation. However, for more casual readers not interested in committing to a long-term paid subscription, paywalls are viewed as an annoyance interrupting their browsing experience. Therefore, paywalls must be effective enough to encourage subscriptions while not overly restrictive to deter potential readers.
Types of paywalls
Since content, publishers, and audiences differ, paywalls must also vary in implementation and restrictions. Paywalls are generally categorized based on how flexible or porous the barriers are between readers and content. Some of the common types used by publishers today include:
Hard Paywalls
The most restrictive of them all, hard paywalls allow no free access to content. Users must subscribe before viewing any articles or content on the site. Media with highly valued and unique content, such as specialized news outlets or educational resources, often use this model.
Metered or Soft Paywalls
Metered paywalls allow casual readers access to a limited number of articles within a certain period before requiring them to sign up for paid access. This model enables publishers to balance accessibility and monetization, offering a taste of content before encouraging avid readers to subscribe for more.
Freemium Paywalls
With freemium paywalls, a publisher makes some content available for free while cordoning off premium content for paying subscribers only. This method allows casual readers basic access while monetizing the most valuable content. Additionally, publishers might offer perks like ad-free viewing to entice readers to subscribe.
Hybrid Models
Some publishers use hybrid paywall models that blend hard, metered, and freemium approaches across content types, devices, or referral sources. The model provides the most flexibility to balance subscription revenue with advertising and sponsored content.
Common reasons for using a paywall
Implementing a content paywall is a strategic decision for many online platforms, with several compelling reasons driving this choice. Publishers should consider their audience, content, and business goals when deciding if paywalls suit them. Some of the common reasons include:
Revenue Generation & Diversification
The most straightforward reason to use a paywall is to generate income directly from content. It's a direct line between content and revenue, making financial returns more predictable and stable. Many content creators, especially in the news and media industry, require more than ad revenues to sustain operations. A paywall offers a more sustainable business model, providing a consistent revenue stream supporting high-value content creation, staffing, and other operational expenses.
Enhanced User Experience
Paywalls can lead to a cleaner, more enjoyable user experience without the need for intrusive advertising. This experience can be a significant draw for users tired of navigating through ads, leading to higher engagement and longer time spent on the platform. Additionally, requiring an account to access content means publishers can build a community or a loyal user base and better understand their readers' preferences. These insights allow them to personalize content and offer even more value to their readers.
Content Valuation
Implementing a paywall places a value on the content, indicating its worth to the audience. This tactic can be particularly effective for niche or specialized content, where users recognize and are willing to pay for quality. Paywalls help distinguish your content from the vast ocean of free online material.
Should you use a paywall?
Deciding on whether to implement a paywall depends on a few key considerations. First, evaluate your content's uniqueness and your audience's willingness to pay. Is your content specialized? If so, a dedicated audience is more likely to pay for exclusive material. Also, consider the competitive landscape: if similar content is widely available for free, you need a strong value proposition to justify a paywall.
Another important aspect is the impact on audience growth. A paywall can restrict the reach of your content, potentially slowing down the expansion of your user base. Soft paywall options, like metered access, offer a balance, allowing some level of free access while monetizing more engaged users.
Financial sustainability is also a key factor. A paywall could provide a more stable income source if ad revenue is insufficient. However, aligning this with your overall financial goals and brand perception is essential. Implementing a paywall positions your content as premium, which can influence how your audience perceives your brand.
Ultimately, deciding to use a paywall depends on carefully analyzing your content's value, audience dynamics, financial needs, and brand strategy.
Are paywalls effective for anonymous users?
Paywalls face a challenge with anonymous users, particularly those using incognito modes and actively trying to evade paywalls. In 2017, DuckDuckGo discovered that 26.7% of surveyed people use incognito mode at least once a week. As online publications shift from display advertising to premium subscriptions, the importance of protecting paid content from private browsing abuse increases. Traditional user identification methods, like cookies, are less effective in these scenarios. However, advanced techniques like device fingerprinting can circumvent this issue by identifying unique characteristics of a user's device, enabling paywall enforcement even for users who attempt to get around your paywall without paying.
What is paywall evasion?
Paywall evasion refers to dubious tactics and workarounds that users use to access paywalled content without paying. These tactics range from simple tricks like clearing browser cookies to more sophisticated techniques involving third-party software and scrapers. Publishers often view these techniques as unethical because they deny the revenue their work rightfully deserves and violate their terms of use.
Some methods, such as disabling JavaScript, exploit loopholes in a website's code, while others use aggregator services that save content to outside databases. Website visitors have devised several methods to bypass paywalls and access gated content for free:
Incognito Mode and Clearing Cookies
Many users access content behind soft or metered paywalls using their browser's incognito or private browsing mode. Since these private windows do not store cookies each session, a website that relies on cookies to track previous visits cannot recognize the user's repeat visits. Similar to using incognito mode, users can manually clear their browser cookies to reset the metered paywall count, allowing them to access more articles for free.
Disabling Javascript or Reader Mode
Many paywalls rely on JavaScript to tally page views and limit non-paying readers. Disabling JavaScript in the browser settings prevents the paywall from loading, bypassing restrictions. Additionally, many modern browsers offer a reader mode that removes ads and other elements from a webpage for easier reading. However, these methods may also affect the website's functionality and lead to a poor user experience.
HTML and CSS Edits
A common method among tech-savvy users involves manipulating the website's CSS using the browser's developer tools. By altering or removing specific styles and elements, users can reveal hidden content behind soft paywalls, bypassing the need for subscription or payment.
Browser Extensions
A less technical approach to paywall evasion is using browser extensions that can perform customized rule-based evasions and automatically do the work for users behind the scenes. These extensions are usually tailored to major media sites and get around paywalls by exploiting loopholes in the website's front-end code. These extensions manipulate cookies or block certain page sections to display only the article content.
Third-Party Systems
Some users turn to third-party services or proxies to circumvent paywalls. These services allow users to save paywalled articles from media sites to outside databases, stripped of the originating domain's paywall. This content is then accessible for free public viewing. Websites like the Wayback Machine or Google's cached pages can provide access to content by displaying saved versions of web pages that might not include the paywall.
Multiple Devices or Browsers
To bypass paywalls, users often switch between different devices or browsers. Each device or browser can be perceived as a distinct user by the website, granting access to more free articles than intended. Some users also utilize multiple devices concurrently, such as a smartphone and laptop, to circumvent the limitations of a metered paywall. However, this strategy is limited to the number of devices they have.
How to reinforce your paywall
Understanding the tactics used by users to evade paywalls is crucial for content providers to develop more robust and effective paywall strategies and stay up-to-date with the latest technology. The paywall is ineffective if visitors can bypass paywalls with a few simple workarounds. This ineffectiveness leads to overuse and unauthorized access to paid content, resulting in lost revenue.
The key is balancing access control and user experience, ensuring your content remains protected and accessible to your legitimate audience. While not wholly bulletproof, publishers can take measures to significantly raise the effort and skill level needed to bypass their paywalls at scale while maintaining a good user experience.
Server-Side Content Delivery
Deliver paywalled content from your back-end only after confirming the visitor is a paying user. This approach adds a layer of security against client-side bypass methods, making accessing premium content more challenging for non-paying users. However, dynamic server-side processing can slow down content delivery.
Device Analysis
Employ advanced device intelligence and fingerprinting techniques to identify users and tally the articles they've accessed. This method can include analyzing device characteristics like the operating system, browser version, and hardware configuration. Device fingerprinting provides a more long-lasting identifier than cookies, which users or private browsing modes can quickly delete.
Dynamic Obfuscation
Implement dynamic obfuscation of website elements to make it harder for users to manipulate the site's CSS and HTML to evade the paywall. Randomizing class names and ID attributes can also make it harder for automated content scrapers to parse your site.
Regular Audits
Conduct regular audits of your paywall system to identify potential vulnerabilities and address them promptly. Watch for any suspicious spikes in traffic that might indicate bulk scraping of your content. Use bot detection software to recognize automated scrapers and prevent bots from scraping your content.
Legal Notices
Communicate the legal implications and ethical considerations of paywall evasion to your users. Highlight the terms of service and the importance of supporting content creators. Additionally, notify third-party services of unlawfully republished content on their sites.
How to protect your paywall with Fingerprint
Fingerprint provides a unique and stable visitor identifier for every visitor on your website. The identifier is based on the browser or device fingerprint and stays the same even when the user switches to incognito mode, deletes their cookies, or turns on a VPN.
Using our visitor identifier in your paywall implementation enables you to prevent non-paying visitors from going around your paywall and push them to subscribe. At the same time, legitimate visitors can get a taste of your content without signing up or facing additional friction. You decide how exactly to use the visitor identifier in your paywall implementation. Below are some steps and best practices to use as a starting point for your solution.
How to implement paywall protection
In this example, we will implement a simple paywall:
- When visitors open an article, save their visitor identifier to your database and increase their view count.
- If their view count exceeds a certain threshold, display a prompt to subscribe instead of the article content.
1. Install the Fingerprint JavaScript agent
First, sign up for a Fingerprint Pro account (we offer a 14-day free trial) and add the JavaScript agent to your website client. We have client libraries for popular front-end frameworks, or you can load the script from our CDN as shown below:
// Initialize the agent.
const fpPromise = import("https://fpjscdn.net/v3/<your-public-api-key>").then(
(FingerprintJS) =>
FingerprintJS.load({
endpoint: "https://metrics.yourdomain.com",
})
);
// Collect browser signals and request visitor identification
// from the Fingerprint API. Typically on page load or on button click.
// The response contains a `requestId`.
const { requestId } = await (await fpPromise).get();
We recommend routing requests to Fingerprint's APIs through your domain for production deployments using the endpoint parameter. This method prevents ad blockers from disrupting identification requests and improves accuracy. We offer a variety of ways to do this, which you can learn more about in our guide on how to protect your JavaScript agent from ad blockers.
2. Send the visitor and request ID to your server
On the client, when a visitor opens an article, send the article identifier with Fingerprint's visitorId
and requestId
to your API.
// Send the `requestId` to your back-end.
const response = await fetch(`/api/paywall/article/${articleId}`, {
method: "POST",
body: JSON.stringify({ requestId }),
headers: {
"Content-Type": "application/json",
Accept: "application/json",
},
});
3. Verify the visitor ID server-side
Do all the following steps on the backend. All client-side code can be tampered with. On the server, it's essential to check the authenticity of the visitor ID received from the client. The example below uses Next.js, but the idea is the same for all server frameworks and languages.
Use the Fingerprint Server API to get the complete identification result using the requestId
. Alternatively, you can also receive the identification event using webhooks. If the requestId
does not have an identification event, it is likely forged. Do not serve content to users who send forged data. You can call the API directly or use one of our backend libraries. This example uses the Fingerprint Server API Node SDK.
import {
FingerprintJsServerApiClient,
Region,
} from "@fingerprintjs/fingerprintjs-pro-server-api";
export default async function checkLoanApplication(req, res) {
const { requestId } = req.body;
let visitorData;
try {
// Initialise the Server API client.
const client = new FingerprintJsServerApiClient({
apiKey: "<YOUR_SERVER_API_KEY>",
region: Region.Global,
});
// Get the identification event from the Server API using the `requestId`.
const eventResponse = await client.getEvent(requestId);
visitorData = eventResponse?.products?.identification?.data;
} catch (error) {
// If getting the event fails, it's likely that the
// `requestId` was spoofed; do not serve the content.
reportSuspiciousActivity(req);
return getForbiddenResponse();
}
// The returned data must have the expected properties.
if (visitorData?.visitorId == undefined) {
reportSuspiciousActivity(req);
return getForbiddenResponse();
}
// Continue with more checks...
}
An attacker might have acquired a previously issued valid requestId
and visitorId
. To prevent replay attacks, check the freshness of the identification request.
// Fingerprinting event must be a maximum of 3 seconds old.
if (new Date().getTime() - visitorData.timestamp > 3000) {
// Do not serve the content.
return getForbiddenResponse(req);
}
The event's confidence score reflects Fingerprint's degree of certainty that the visitor identifier is correct. If it's lower than a certain threshold, we recommend not to serve content.
// The Confidence Score must be of a certain level.
if (visitorData.confidence.score < 0.95) {
// Do not serve the content.
return getForbiddenResponse();
}
Check if the content request comes from the same IP address as the identification request.
// This is an example of obtaining the client's IP address.
// In most cases, it's a good idea to look for the right-most
// external IP address in the list to prevent spoofing.
if (request.headers["x-forwarded-for"].split(",")[0] !== visitorData.ip) {
// Do not serve the content.
return getForbiddenResponse();
}
Verify that the identification request's origin matches the content request's origin. Usually, both will be coming from your website's domain. Additionally, you can set up Request Filtering in the dashboard to filter out requests from unknown origins.
const ourOrigins = ["<https://example.com>"];
const visitorDataOrigin = new URL(visitorData.url).origin;
// Confirm that the purchase request is from a known origin.
if (
visitorDataOrigin !== request.headers["origin"] ||
!ourOrigins.includes(visitorDataOrigin) ||
!ourOrigins.includes(request.headers["origin"])
) {
// Do not serve the content.
return getForbiddenResponse();
}
4. Implement your paywall using the visitor ID
Eventually, apply your paywall policy for free content. As in the example below, you can record access to content for a given visitorId
daily.
// Saves article view into the database. If it already exists,
// we update its timestamp.
export async function saveArticleView(articleId, visitorId) {
const { timestampEnd, timestampStart } = getTodayDateRange();
const [view, created] = await ArticleView.findOrCreate({
where: {
articleId: {
[Op.eq]: articleId,
},
visitorId: {
[Op.eq]: visitorId,
},
timestamp: {
[Op.between]: [timestampStart, timestampEnd],
},
},
defaults: {
articleId,
visitorId,
timestamp: new Date(),
},
});
if (!created) {
view.timestamp = new Date();
await view.save();
}
return view;
}
If the visitor exceeds their free quota, don’t serve any additional content.
// Checks if the given visitor has exceeded their daily limit
// of free article views.
export async function checkCountOfViewedArticles(visitorId, req) {
const { timestampEnd, timestampStart } = getTodayDateRange();
const [count, existingView] = await Promise.all([
countViewedArticles(visitorId),
ArticleView.findOne({
where: {
visitorId: {
[Op.eq]: visitorId,
},
articleId: {
[Op.eq]: req.query.id,
},
timestamp: {
[Op.between]: [timestampStart, timestampEnd],
},
},
}),
]);
if (!existingView && count >= ARTICLE_VIEW_LIMIT) {
return new CheckResult(
"You have reached your daily view limit, purchase our membership plan to view unlimited articles.",
messageSeverity.Error,
checkResultType.ArticleViewLimitExceeded
);
}
}
Go beyond visitor identification with Smart Signals
Besides the visitor identifier, the Fingerprint Pro Plus plan provides a variety of other signals that help you outsmart fraudsters concealing their identity. For example, you can detect if the visitor is in incognito mode, tried to tamper with their browser configuration, or is connected to the website using a VPN. You can use Smart Signals as additional data points for your paywall implementation. Read our Smart Signals blog to learn more.
Explore our paywall demo
We have built a Paywall demo to demonstrate the concepts above. Explore using Fingerprint to manage anonymous visitors and their access to your paywalled-content. You can examine the open-source code on Github or run it in your browser with CodeSandbox.
If you have any questions, please get in touch with our sales team or start a 14-day free trial.
FAQ
Determining the most effective type of paywall for a particular type of content or audience can be a complex process, often requiring a strategic approach. Businesses should consider factors such as the uniqueness and value of their content, the demographics and behavior of their audience, and their overall business goals. For example, if the content is highly unique and valuable, a hard paywall might be suitable. On the other hand, if the audience is largely casual readers who may not be willing to pay, a metered paywall or freemium model might work better. It's also crucial to conduct market research and A/B testing to make informed decisions.
Customer reactions to different types of paywalls can vary significantly, which can greatly impact user experience and engagement. Some users might be willing to pay for high-quality, exclusive content, thereby responding positively to hard paywalls. However, others might find paywalls off-putting and decide to seek free alternatives elsewhere, particularly if they encounter a paywall unexpectedly. Therefore, it's essential to communicate clearly about the paywall and provide sufficient free content or trials to demonstrate the value of the paid content.
Implementing a paywall can present several potential challenges for businesses. One of the primary concerns is the risk of alienating users who are unwilling or unable to pay, which could lead to a decrease in site traffic and engagement. There's also the technical aspect of setting up a secure and efficient payment system, protecting against bypass attempts, and ensuring a smooth user experience. Moreover, businesses need to continuously produce high-quality content that justifies the cost, which can require significant resources and efforts.
A paywall restricts access to content, requiring users to pay for full access. It can be hard (blocking all content), soft (allowing some free content), or metered (limiting the number of free articles).
First start by analyzing your audience and content type. Metered paywalls work well for news sites wanting to offer some free articles, while hard paywalls suit exclusive, high-quality content providers.