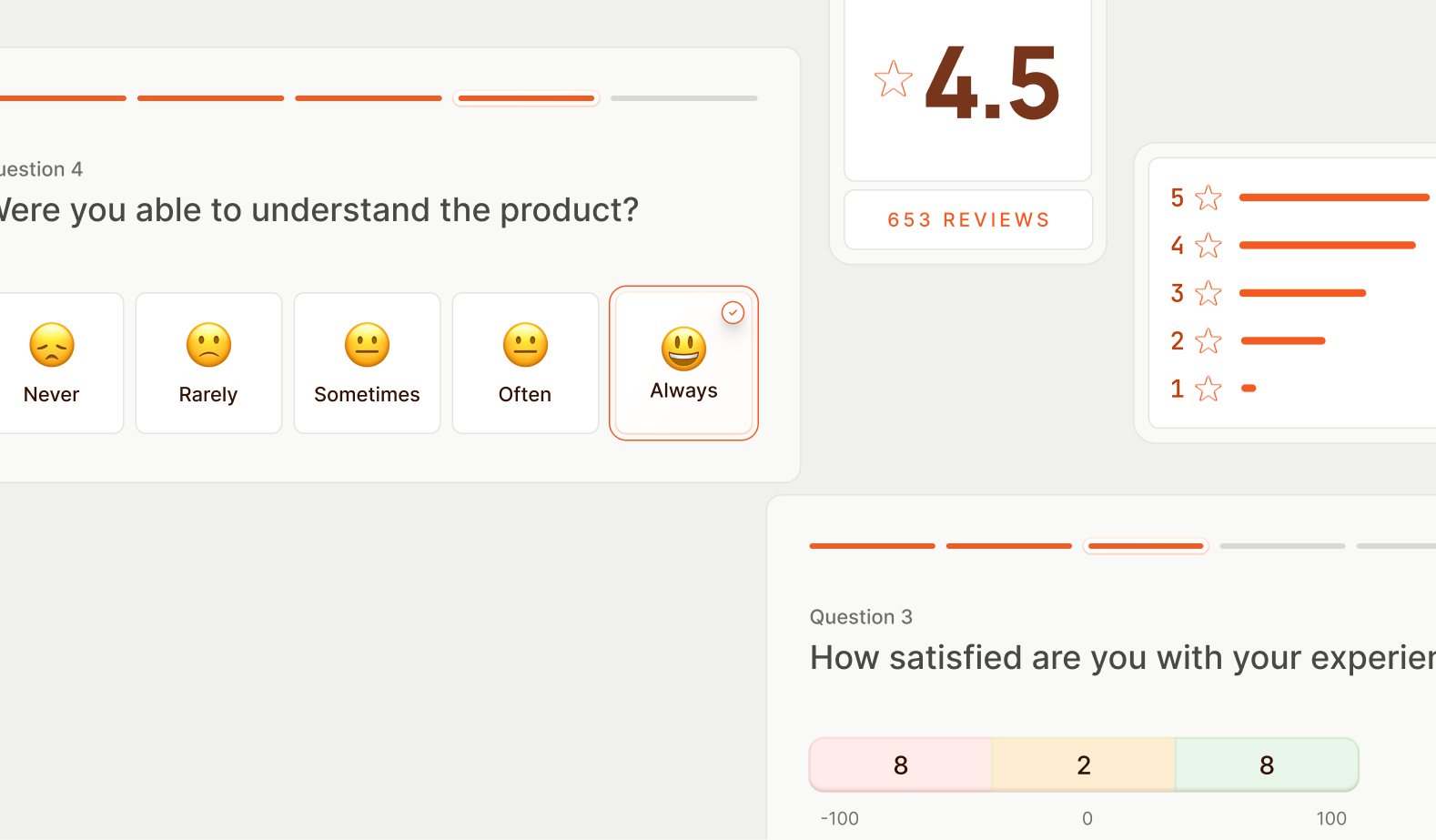
We all rely on reviews on the internet, whether we’re deciding what brand of laundry detergent to buy or where to go out for a celebratory dinner. But how many of those glowing ratings or 1-star complaints are from real people — and how many are generated by bots?
Review fraud is a widespread issue. In 2023:
- TripAdvisor took down 8.8% of reviews on its platform deemed fake
- Google blocked over 170 million fake reviews worldwide
- Amazon removed over 250 million fake reviews
The good news is, you don’t have to be Google or Amazon to implement strategies that prevent fake reviews from harming your business. In this article, you’ll learn how you can fight review fraud with Fingerprint.
What is review fraud?
Review fraud is exactly what it sounds like: fake reviews designed to influence the reputation of a product, service, or company. There are two flavors:
- Fake positive reviews, where fraudsters purchase or generate five-star ratings — most often, to boost their own rankings and sales
- Fake negative reviews, where fraudsters try to use low ratings to hurt a product or service, likely a competitor (when this happens in large numbers, it’s called “review bombing”)
The impact of review fraud on consumers + online marketplaces
By some estimates, fake reviews influence over $152 billion in global online spending. Reviews hurt consumers by misleading them into wasting money and time on subpar goods. Even worse, fraudsters sometimes use fake reviews to execute phishing attacks or redirect visitors to counterfeit products.
For e-commerce marketplaces, the costs of review fraud are high. They include operational costs from customer complaints, returned items, regulatory fines, and even litigation. There’s also the loss of trust that’s harder to measure, but equally impactful. If a customer receives a product or service that doesn’t match the five-star reviews, they’re less likely to return to make another purchase — and they might also tell other people not to shop there. Fake reviews damage your brand reputation and lead to revenue loss over time.
How review fraud happens
There’s a thriving underground economy for review fraud. While some fake reviews are left by real people (who are paid a commission to purchase the item and leave a 5-star review), many others are generated by bots.
It’s not complicated to write a script that can create a new account and post a review — then rinse and repeat. Even when all these accounts come from the same source device, fraudsters can avoid detection by using VPNs to change their IP addresses, clearing cookies, or tampering with their browser settings. More sophisticated fraudsters even use CAPTCHA-solving services to bypass anti-bot protections. They can also steal or spoof order details, or practice refund abuse, to make it seem as if they purchased the product legitimately.
Review fraud is also getting a boost from AI, which can output unlimited numbers of realistic-sounding reviews at scale. It can even tailor content based on typical review patterns or the specific product being reviewed.
Ways to fight review fraud
You can fight review fraud two ways: by finding and removing fake reviews after they’ve been posted, and by stopping real and automated accounts from posting in the first place. Both strategies are necessary in the cat-and-mouse game of staying one step ahead of fraudsters.
Auditing reviews and looking for signals that indicate fraud involves a combination of automation (to flag suspicious reviews at scale) and manual effort (to avoid accidentally penalizing real reviewers). To flag reviews, many companies use a combination of sentiment analysis, where models analyze the writing quality or content, and behavioral analysis, where models look at account usage patterns. Marketplaces may also allow customers to flag suspicious reviews.
While these methods are necessary to catch some of the sneakiest forms of review fraud (like paying real people to write positive reviews), the downside is that they often introduce a delay. One study foundthat Amazon takes around 100 days to pull down fake reviews.
To prevent accounts from posting in the first place, websites have a few options: They can introduce friction into the review process, such as requiring customers to provide proof of purchase or prove they are real humans by completing annoying CAPTCHAs — but, as mentioned previously, sophisticated fraudsters can find ways around these roadblocks. Additionally, marketplaces can use device intelligence to understand visitor behavior and detect suspicious reviews and bot activity without frustrating legitimate customers.
Fingerprint device intelligence
When it comes to detecting suspicious or bot-driven behavior before reviews are made, Fingerprint can help. Fingerprint device intelligence can create a unique digital fingerprint, a visitor identifier, for online browsers and devices accessing your website or application. This visitor ID can be used to detect suspicious devices or behavioral patterns in real time. Fingerprint analyzes over 100 device attributes like browser configurations, operating system details, and hardware settings to recognize returning visitors with industry-leading accuracy.
Fingerprint Smart Signals goes one step further and provides actionable insights about your visitors. For example, it can detect if a visitor is using a VPN, has tampered with their browser, or is a bot. Fingerprint also provides a “Suspect Score” for each device that companies can use to assess the risk associated with individual site visitors. Based on the information from Smart Signals, businesses can detect suspicious visitors and request additional verification steps while maintaining an uninterrupted experience for legitimate customers.
Detecting review fraud with Fingerprint
In the following examples, we’ll show how you can use Fingerprint to detect suspicious account creation patterns, stop known offenders from posting reviews, and flag potential bots and risky visitors, all with just a few lines of code. To use these code snippets on your website, first create a Fingerprint account and open your API keys page. Then, customize and embed the following scripts into the appropriate areas of your website.
Note: If you’re following along and using an ad blocker, your implementation may not work. Simply turn it off while testing, and learn more about how to protect your production Fingerprint implementation from ad blockers in our documentation.
1. Detect suspicious account creation patterns on the same device
In this first part, you will learn how to use Fingerprint to detect suspicious account creation patterns associated with review fraud, such as creating multiple accounts in a short time frame from the same device. Similar logic can be used to detect a single device accessing multiple accounts even if they did not create them.
To get started, add this code to the pages you want to protect. Replace PUBLIC_API_KEY
with the public API key shown on the API keys page:
<script>
const fpPromise = import('https://fpjscdn.net/v3/PUBLIC_API_KEY')
.then(FingerprintJS => FingerprintJS.load());
</script>
This is using the CDN approach, which is the easiest for a tutorial. However, feel free to take a look at our available SDKs, which work with plain JS and most modern frameworks.
Let’s assume your page has a form with an email input field and a submit button. You want to protect this form from suspicious account creation patterns with Fingerprint. Here is the code you would add to the page so that when the button is clicked, we can provide the backend with additional information about the visitor:
<script>
document.querySelector('#create-account').addEventListener('submit', async (evt) => {
evt.preventDefault();
const fp = await fpPromise;
const result = await fp.get();
const requestId = result.requestId;
const email = document.querySelector('#email').value;
// Send the requestId along with the request you want to protect from suspicious behavior
fetch('/saveemail', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ requestId: requestId, email: email }),
});
});
</script>
The requestId
is created on the Fingerprint servers and returned each time fp.get()
is called. Our databases record each request and its corresponding visitorId
, which identifies the device.
You can now use the requestId
on the backend to get information about this visitor from the Fingerprint service.
Here is an example of a backend written in Node.js. It will process the form submission, but it always accepts the request given by the front-end code:
const express = require('express');
const app = express();
app.use(express.json());
app.post('/saveemail', (req, res) => {
// Code to save email to database...
res.sendStatus(200);
});
app.listen(3000, () => {
console.log('Listening on port 3000');
});
Let’s protect this code using Fingerprint.
Go to the API keys page and create a secret key. Then, create an environment variable named SERVER_API_KEY
for that key.
In your Node.js app, install the Fingerprint Server API NPM package:
npm install @fingerprintjs/fingerprintjs-pro-server-api
Append the following to the top of your server code:
const {
FingerprintJsServerApiClient,
Region,
} = require('@fingerprintjs/fingerprintjs-pro-server-api')
const client = new FingerprintJsServerApiClient({
apiKey: process.env.SERVER_API_KEY
region: Region.Global,
})
Replace your saveemail
route with this code:
app.post('/saveemail', async (req, res) => {
const { requestId } = req.body;
const event = await client.getEvent(requestId);
const visitorId = event.products.identification.data.visitorId;
// Check how many times this visitor has created an account
const res = await client.query('select count(*) as count from account where created > NOW() - INTERVAL '30 DAY', visitorid = $1, [visitorId])
If (res.rows[0].count > 2) {
req.sendStatus(403);
return;
}
// Code to save email and visitorId to database...
res.sendStatus(200);
});
// ... as before ...
Whenever an account is created, store the visitorId
with that account. This means before a new account is created, we can check how many times that visitorId
has been used to create a new account in the last 30 days.
If a fake requestId
is sent, this will not help someone create additional accounts. In this case, the getEvent
method will throw an error, stopping the account from being created.
2. Recognize known offenders and prevent new reviews
Fraudsters will often use the same device for review fraud, meaning businesses can take action against known offenders at the device level. The following example shows how Fingerprint can be used to block a device from posting new reviews, regardless of which account it’s posting from.
We will adapt the code from the previous example to work for this scenario. In this case, the form is likely to have different fields, such as reviewText
and starRating
instead of email
. However, we will still initialize the script and post the requestId
to check on the backend if we recognize the device as being used by a known offender.
To detect known offenders, we can create a database table called blocked_visitors
that stores the blocked visitorId
. In addition, we can store visitorId
along with every review. Whenever a site admin sees a spam review, they press a button that hides that review and adds the visitorId
to blocked_visitors
.
Here is what the code would look like, for handling the posting of a new review.
app.post('/postreview', async (req, res) => {
const { requestId } = req.body;
const event = await client.getEvent(requestId);
const visitorId = event.products.identification.data.visitorId;
// Check if this visitor is blocked
const res = await client.query('select count(*) as count from blocked_visitors where visitorid = $1, [visitorId])
If (res.rows[0].count >= 1) {
// The browser can handle this by either informing the user, or
// claiming the comment was posted and is being reviewed (which
// is called shadow-banning). If shadow banning, you could even
// return 200 success here.
req.sendStatus(403);
return;
}
// Code to save comment and visitorId to database...
res.sendStatus(200);
});
3. Use Smart Signals to determine suspicion
The following example demonstrates how Fingerprint Smart Signals can detect bots or score the level of risk associated with a visitor, which can help businesses block malicious devices and fraudsters. Using the information from Smart Signals, businesses can automatically introduce friction (e.g., one-time passcodes) to slow down risky visitors and stop bots.
Returning to our signup form example, we will now use Smart Signals and require an SMS verification if the request looks suspicious. Here is some example code to do this:
app.post('/saveemail', async (req, res) => {
const requestId = req.body.requestId;
// A token provided by the smsVerification flow once successful
const smsVerificationToken= req.body.smsVerificationToken;
const event = await client.getEvent(requestId);
let verificationSuccess = false;
// ... existing code to check if visitorId has been used before ...
if (smsVerificationToken) {
// isVerificationTokenCorrect checks if the SMS verification has been successfully completed
verificationSuccess = await isVerificationTokenCorrect (smsVerificationToken);
}
if (!verificationSuccess) {
const botDetection = event.products.botd.data.bot.result;
if (botDetection != "notDetected") {
res.json({ result: "error" });
}
const suspectScore = event.products.suspectScore.data.result;
if (suspectScore > 15) {
res.json({result: "requiresSMSVerification",});
return;
}
}
// Code to save email and visitorId to database...
res.json({"result":"success"});
});
This code uses Bot Detection and Suspect Score signals. The Bot Detection signal returns good
for known bots like search engines, bad
for potentially malicious bots, or notDetected
when no bot is detected. The Suspect Score looks at a wide range of signals to determine how suspicious the request is, including VPN usage, incognito mode, IP, Tor exit nodes, and many other factors.
The UI will need to now redirect to an SMS verification flow if requiresSMSVerification
is returned. Once that flow is complete, it will get a token that it can include in the form.
Fight review fraud with device intelligence
Review fraud is everywhere, and it causes real harm to e-commerce customers and businesses. But as fraudsters evolve their tactics, there are also more choices for businesses that want to fight review fraud today. With Fingerprint’s device intelligence and Smart Signals, you can flag suspicious account creation patterns, prevent new reviews at the device level, and introduce friction when bots or suspicious behavior is detected.
Want to learn more about how Fingerprint can protect your business? Sign up for a free trial or contact our sales team for more information.